Table of Contents
Introduction: The Art of Building Scalable Apps
The world runs on high-performance applications—whether it's Netflix streaming billions of hours, Uber handling millions of rides, or Instagram processing endless photos. But what makes these apps scalable, secure, and superfast?
Scalability is not just about adding more servers but about designing systems that efficiently handle increasing loads without compromising performance or security. This requires a combination of solid architecture, database optimization, caching mechanisms, load balancing, and backend efficiency.
In this guide we'll walk through the engineering principles and battle-tested strategies that help businesses scale from their first few users to handling millions. By the end you'll have a practical blueprint for building systems that scale effortlessly.
System Design: Architecting for Scale
Scalability begins at the architectural level. Choosing the right structure, handling traffic efficiently, and designing an API that can withstand high loads are fundamental to building a system that can scale effortlessly.
There are various architectural patterns for structuring applications but in this guide we'll only look at the two most popular among them. The Monolith and the Microservices pattern.
Monolith Architecture
A monolith is a single application where all components (UI, business logic, database, etc.) are combined in one codebase and deployed as a single unit.
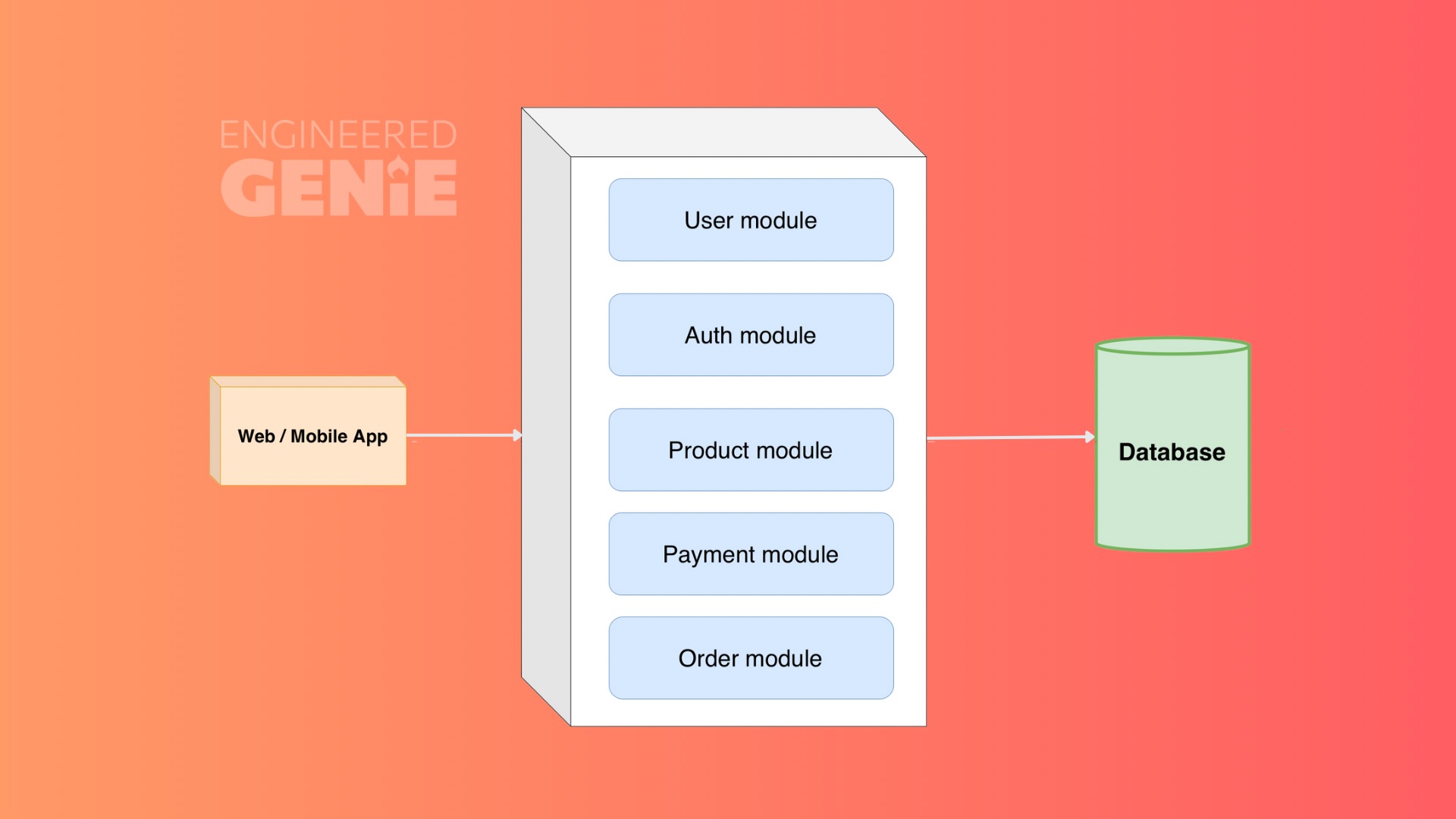
Example: E-commerce in Monolithic Architecture
Imagine you are building an online store where users can:
- View products (Product Management)
- Add to cart and place orders (Order Management)
- Make payments (Payment Processing)
In a monolith architecture all the modules are tightly bound to each other and connects to a single database.
Pros of Monolith Architecture
- Easy Development: Everything is in one place, so it's easier to start.
- Simpler Deployment: Only one application to deploy requiring only a single CI/CD pipeline.
- Easier Debugging: Logs are centralized, making issues easier to track.
Cons of Monolith Architecture
- Scalability Issues: If one feature (e.g payments) gets high traffic, the entire app needs scaling.
- Slower Development Over Time: As the app grows, making changes becomes harder.
- Single Point of Failure: A bug in one module (e.g payments) can crash the entire app.
Use Cases
You should prefer monolith architecture when building small scale apps, single team development, low user base internal applications, and small to medium sized apps.
Microservice Architecture
Microservices architecture breaks down an application into independent, loosely coupled services that communicate via APIs like REST, GraphQL, gRPC, Kafka or any other transporters.
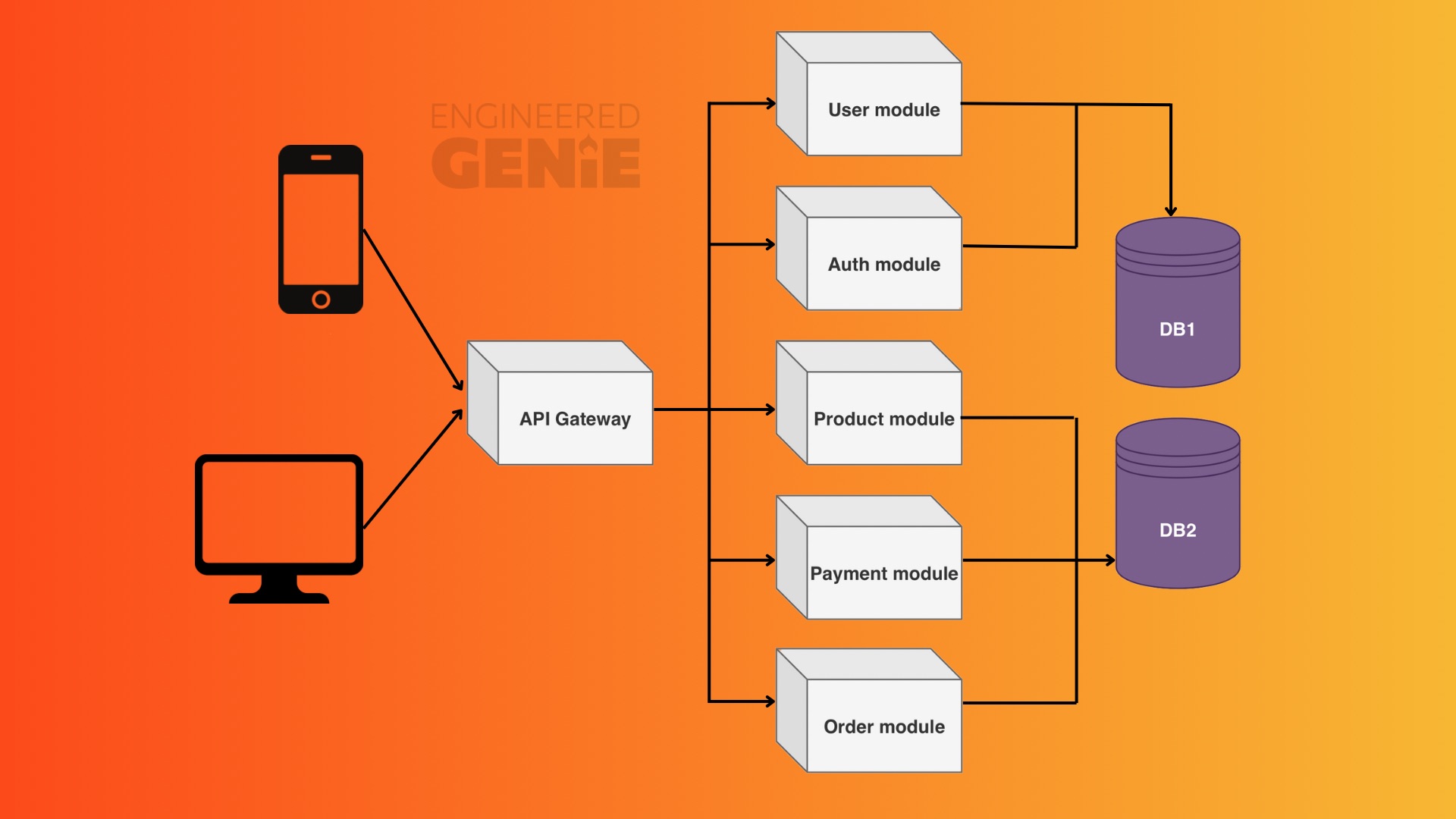
Let's consider the same set of processes managed in a monolithic architecture and define them as microservices. In this approach, each module is set up as a separate project or within a monorepo to utilize shared libraries efficiently. This allows each module to connect to its own database, providing greater flexibility.
Pros of Microservice Architecture
- Scalability: Services can scale independently. For example, if search function is heavily utilized only the search service scale separately from the rest.
- Polyglot: Each service can be implemented using a different technology like Python for efficient reporting, NodeJS for handling huge traffic and Java for CPU intensive tasks.
- Faster Development: Teams can work independently allowing parallel work and avoiding conflicts.
- Fault Isolation: A failure in one service does not crash the entire system.
Cons of Microservice Architecture
- Complex Setup/Deployment: Each service needs to be maintained and deployed separately. However, with CI/CD tools this can be automated and proven beneficial in the long run.
- DevOps Team: DevOps engineers are required to setup containers and orchestrate them for scaling and manage infrastructure.
- Harder Debugging: Application logs are spread across multiple microservices. Developers needs to spend extra time to monitor the logs alone.
Monolith vs Microservice
Feature | Monolithic | Microservices |
---|---|---|
Architecture | Single codebase | Multiple independent services |
Scaling | Scale the whole app | Scale individual services |
Technology | One tech stack | Different stacks for different services |
Deployment | One deployment | Deploy services independently |
Maintenance | Harder as the app grows | Easier to maintain smaller services |
Failure Impact | One crash affects the entire app | Isolated failures per service |
Use Case | Best for small to medium apps | Best for large, scalable apps |
Which architecture is appropriate for your project?
As the pioneers say, there is no definitive THIS or THAT answer when it comes to architectural patterns. The right choice depends on your application's requirements, user base, company's tech stack, and team size. Netflix was one of the first to transition from a monolithic architecture to a microservices-based approach to accommodate its growing needs. They started small, scaled significantly, and found that the microservices pattern was a game-changer for their expansion.
Now that you're familiar with architectural patterns, let's move on to Load Balancing and Traffic Distribution.